
For example, If you want to store the name and roll number of all students, then you can use the dict type. The dictionary type is represented using a dict class. Use a dictionary data type to store data as a key-value pair.
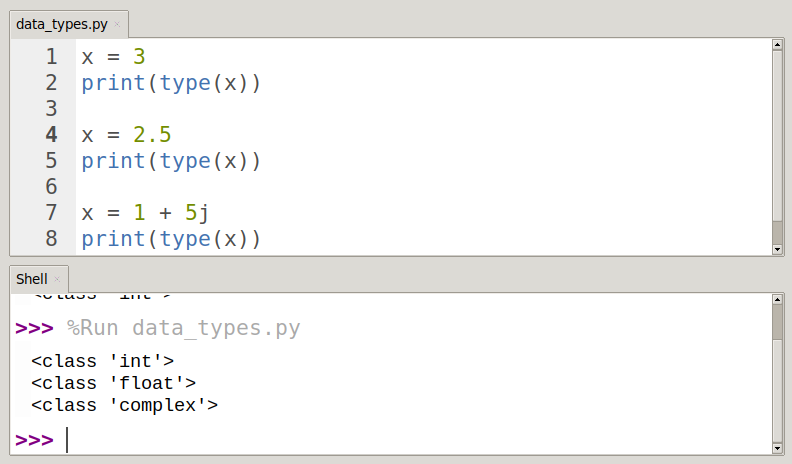
In Python, dictionaries are unordered collections of unique values stored in (Key-Value) pairs. # TypeError: 'tuple' object does not support item assignment Dict data type By enclosing elements in the parenthesis ()Įxample Tuple creation and manipulation # create a tupleĪ tuple is immutable means once we create a tuple, we can’t modify it # create a tuple.Note: Tuple maintains the insertion order and also, allows us to store duplicate elements. In other words, we can say a tuple is a read-only version of the list.įor example: If you want to store the roll numbers of students that you don’t change, you can use the tuple data type. The tuple is the same as the list, except the tuple is immutable means we can’t modify the tuple once created. Tuples are ordered collections of elements that are unchangeable. Įxample list creation and manipulation my_list = By enclosing elements in the square brackets.The list is mutable which means we can modify the value of list elements.Duplicates elements are allowed in the list.The list can contain data of all data types such as int, float, string.

For example: If we want to store all student’s names, we can use list type. We use the list data type to represent groups of the element as a single entity. List elements can be accessed, iterated, and removed according to the order they inserted at the creation time. The Python List is an ordered collection (also known as a sequence ) of elements. If we are trying to represent an imaginary part as binary, hex, or octal, we will get an error. But the imaginary part should be represented using the decimal form only. The integer value can be in the form of either decimal, float, binary, or hexadecimal.

The real part of the complex number is represented using an integer value. Z = 11.2 + 1.2j # both value are float type Example x = 9 + 8j # both value are int type If we want to declare a complex value, then we can use the a+bj form. The complex type is generally used in scientific applications and electrical engineering applications. Print(type(num1)) # class 'float' Complex data typeĪ complex number is a number with a real and an imaginary component represented as a+bj where a and b contain integers or floating-point values. The benefit of using the exponential form to represent floating-point values is we can represent large values using less memory. # store a floating-point value using float() classįloating-point values can be represented using the exponential form, also called scientific notation. Directly assigning a float value to a variable.We can create a float variable using the two ways The float type in Python is represented using a float class. For example, if we want to store the salary, we can use the float type. To represent floating-point values or decimal values, we can use the float data type. Print(type(binary_num)) # class 'int' Float data type Print(type(hexadecimal_num)) # class 'int'īinary_num = 0b10000 # decimal equivalent of 6 Hexadecimal_num = 0x10 # decimal equivalent of 21 You can also store integer values other than base 10 such as Directly assigning an integer value to a variable.
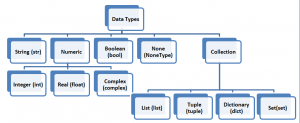
We can create an integer variable using the two ways You can store positive and negative integer numbers of any length such as 235, -758, 235689741. The Integer type in Python is represented using a int class. For example, we can use the int data type to store the roll number of a student. Python uses the int data type to represent whole integer values. # Gives TypeError: 'str' object does not support item assignment Int data type # Now let's try to change 2nd character of a string. This non-changeable behavior is called immutability. You need to create a copy of it if you want to modify it. Note: The string is immutable, i.e., it can not be changed once defined. Once a string is created, we can do many operations on it, such as searching inside it, creating a substring from it, and splitting it. To work with text or character data in Python, we use Strings. The string type in Python is represented using a str class. These characters could be anything like letters, numbers, or special symbols enclosed within double quotation marks. In Python, A string is a sequence of characters enclosed within a single quote or double quote. To store complex numbers (real and imaginary part) In this article, we will learn the following Python data types in detail.
